MongoDB Development: A Complete Guide
Tuesday, Apr 8, 2025
9 min read
Aysham Ali
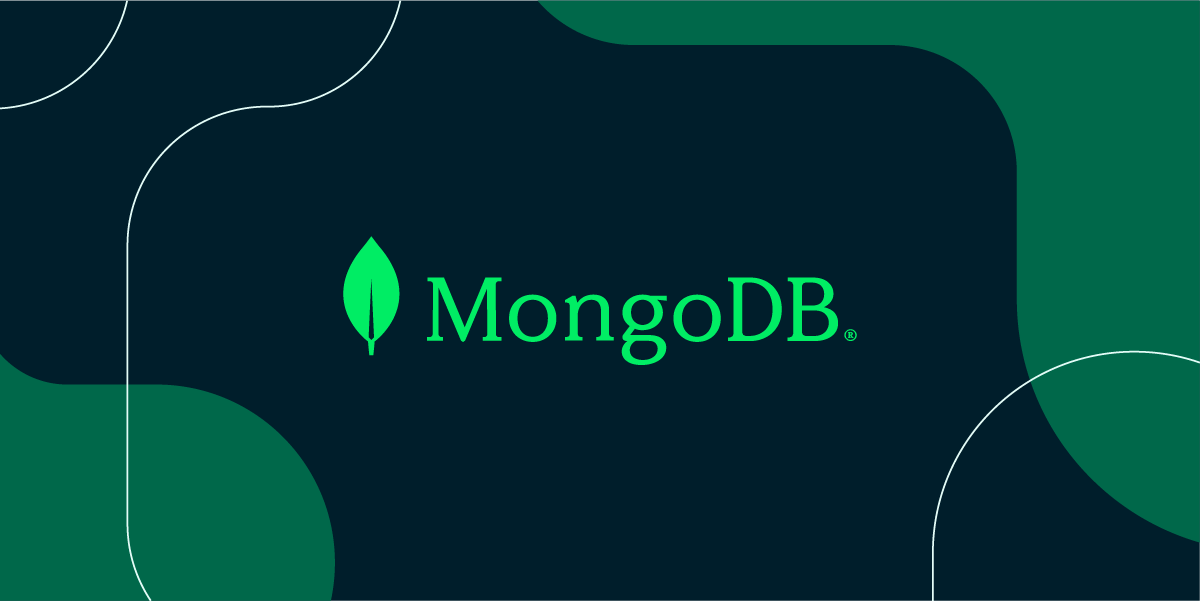
Introduction
What is MongoDb?
It is an open-source, document-oriented NoSQL database. Unlike traditional relational databases that store data in tables and rows. MongoDB stores data in flexible JSON-like documents. This makes it ideal for handling complex, unstructured, and rapidly evolving data.
Main Differences
Relational | MongoDb |
Store data in a table in rows and columns. Each row represents a related data entity, and each column stores an information for that data entity. | Stores data in collections. Each collection is a collection of documents, which stores data in a form of key-value pair. This key-value pair is called a field |
Each table has a predefined schema and while inserting data in the table we have to follow the schema | There is no predefined schema. Two or more documents can have different fields and different number of fields. |
A table can have related data stored in other tables with primary key, foreign key relation. This reduces data redundancy. | Can store related data in the same document. Makes querying related data faster. But might also lead to redundant data. |
MongoDB Documents
A document of a collection stores data in a JSON like format. This format is called BSON
A collection is schema less. Can store 2 different types of data with different fields and different number of fields in the same collection.
Can also store related data of a document in the same document
Document vs Collection
Feature | Document | Collection |
Definition | A single JSON-like record | A group of documents |
Equivalent in SQL | Row | Table |
Structure | Flexible (schema-less) | Can contain different document structures |
Storage Format | BSON (Binary JSON) | Stores multiple BSON documents |
Installation & Configuration
Download MongoDB
Go to https://www.mongodb.com/
Download community edition under products page
Installation
Leave everything default and install it using the wizard.
Configure environment variables
Add C:\Program Files\MongoDB\Server\8.0\bin to system path variable
Installing MongoDB Shell
Go to this website https://www.mongodb.com/try/download/shell
Run this command in cmd to run this shell
mongosh
Starting MongoDB Server Manually on Different Port (Optional)
mongod —dbpath “data folder path here” —port 3000
mongosh —port 3000
To shutdown server in mongosh
use admin
db.shutdownServer()
Understanding Documents
Data Types
Integer | String | Boolean | Array | Object | Null | Date | ObjectId | Binary Data | Regex | Double | etc..
Advantages of Document
Flexibility - Fields can differ across documents in the same collection
Embedded Relationships - allows storing related data together in one document
Rich Queries - provides powerful query operators to work with document structures
Ease of Use - JSON-like syntax is intuitive for developers familiar with JavaScript and JSON
Limits of Document
Size Limit - cannot exceed 16mb in size. If larger, should use GridFs for storing large files
Schema Management - lack of enforced schema can lead to inconsistent data if not carefully managed
Summary
Open source document db |
Written in C++ |
NoSQL Db |
High Availability (database remains accessible even if failures or server crashes ) |
High Performance |
Can handle large scale data |
Relational vs MongoDb Comparison
Relational | MongoDB |
Database | Database |
Table | Collection (No Schema) |
Row | Document |
Column | Field |
Table Join | Embedded Documents |
Using an Editor on Mongosh
To use the built-in mongosh editor
.editor
To use a different editor like for example vscode
Go to environment variables
Under user variables, click new
Add these
Restart cmd
Launch editor using
edit
Working with MongoDB Database
Check existing databases
show dbs
Creating database
use sampledb
Creating a document
db.users.insertOne({name:'John', age:28})
Fetching all documents
db.users.find()
Fetching a document based on criteria
db.users.find({name:'John'})
Dropping database
db.dropDatabase()
The ID field
Each document stored in a collection requires a unique id fields that acts as a primary key. In no id is find inside a document, MongoDB driver automatically generates an ObjectId for the _id field
Providing own Id
db.users.insertOne({_id:123456789,name:'Sam', age:20})
Counting Documents (rows)
db.users.countDocuments()
Querying
Finding (same as where statement) & condition
db.inventory.find({category:'Electronics'})
Finding (multiple where statement) & condition
db.inventory.find({category: {$in: ["Electronics","Accessories"]} })
Same as
select * from inventory where category in (“Electronics”,”Accessories”);
Finding with multiple fields & condition
db.inventory.find({
category: 'Electronics',
price: 25.99
})
Finding multiple fields with lt, gt, gte, lte, ne, nin
db.inventory.find({
category: 'Electronics',
price: {
$gt: 20
}
})
Greater Equal To (gte)
db.inventory.find({
category: 'Electronics',
price: {
$gte: 25.99
}
})
Example of nin (not in)
db.inventory.find({
category:{
$nin: ["Electronics"]
}
})
For more condition examples checkout
Query on Nested Field
Example of nested object:
{
_id: ObjectId('67fe5df261f3c997d0b5f89b'),
item_id: 'SKU67890',
name: 'Mechanical Keyboard',
description: 'Backlit mechanical keyboard with blue switches.',
category: 'Electronics',
quantity: 85,
price: 79.99,
supplier: {
name: 'KeyGear Co.',
contact: 'sales@keygear.com',
phone: '+1-800-987-6543'
},
location: { warehouse: 'B2', shelf: 'C1' },
last_updated: { '$date': '2025-04-12T14:30:00Z' }
},
Finding it (needs exact sequence)
db.inventory.find({
supplier: {
name: "KeyGear Co.",
contact: "sales@keygear.com",
phone: "+1-800-987-6543"
}
})
Searching using Field name
Don’t need to specify whole object for finding
db.inventory.find({
"supplier.name" : "KeyGear Co."
})
Finding an array that contains elements
db.inventory.find({
tags: {
$all: ["red"]
}
})
Finding an array that contains exact elements
db.inventory.find({
tags: ["red", "blue"]
})
Query and Array by Array Length
For example get all the documents that have 3 tags
db.inventory.find({tags: {$size:3}})
Query using index
Get all documents in which tags array at index 0 (first) is blue
db.inventory.find({
"tags.0" : "blue"
})
Displaying certain fields only
db.inventory.find({}, {name:1, description:1}}
Hiding id
db.inventory.find(db.inventory.find({}, {name:1, description:1, _id:0}}
Excluding certain fields
Not displaying _id
db.inventory.find({}, {_id:0}}
Displaying embedded fields only
Get all documents that have 3 tags and display only tags and hide id
db.inventory.find({tags:{$size:3}},{tags:1,_id:0})
Cursors
A cursor in MongoDD is an object that points to the result set of a query. Think of it like a pointer or iterator that allows you to traverse over documents returned from a find() query one-by-one or in batches.
Why do cursors exist?
If the result is huge, MongoDB give you a cursor so you can:
Iterate over results efficiently
Avoid loading too much data in memory at once
Stream large result sets piece by piece
Example in JS
consts cursor = db.users.find({age: {$gt: 25}});
cursor.forEach(user=>{
console.log(user.name);
});
Cursor Behavior
Lazy-loading - fetches data in batches (default is 101 documents in MongoDB shell)
Timeouts - automatic timeouts after 10 minutes of inactivity (unless you prevent that with noCursorTimeout)
Exhausted - once all iteration is done, it closes automatically
Cursor Methods
.toArray() | Converts all documents to an array |
.forEach() | Iterates over each document |
.next() | Gets the next document |
.hasNext() | Checks if more documents are available |
.limit(n) | Limits the number of results |
.skip(n) | Skips the first n results |
.sort({}) | Sorts the result set |
Real world example: db.posts.find().skip(20).limit(10);
Updating Documents
Update a Single Document
db.students.updateOne(
{ name: "Ali" },
{ $set: { age: 23 } }
);
Update Multiple Documents
Updates all documents that match the filter.
db.students.updateMany(
{ major: "Physics" },
{ $set: { major: "Astrophysics" } }
);
Replace One
Replaces entire document (not just field)
db.students.replaceOne(
{ name: "Ahmed" },
{ name: "Ahmed", age: 29, major: "Statistics" }
);
Update Operators
$set | Sets a field’s value |
$inc | Increments a numeric field |
$unset | Removes a field |
$rename | Renames a field |
$mul | Multiplies a field |
$min/$max | Updates field if new value is smaller/larger |
Upserts: Update or Insert
Can tell mongodb to insert a new document if non is found using upsert:true
db.students.updateOne(
{ name: "Imran" },
{ $set: { age: 27, major: "Engineering" } },
{ upsert: true }
);
Get updated document back after updating:
db.students.findOneAndUpdate(
{ name: "Ali" },
{ $set: { age: 24 } },
{ returnDocument: "after" }
);
Deleting
Deleting One
Deletes the first document that matches the filter.
db.students.deleteOne({ name: "Ali" });
Deleting Many
Deletes all documents that match the filter.
db.students.deleteMany({ major: "Physics" });
Deleting All Documents
To wipe the entire collection:
db.students.deleteMany({});
Drop the Collection Entirely
If you want to delete all data and the collection itself:
db.students.drop();
Delete by _id
db.students.deleteOne({ _id: ObjectId("642eabc1234abc567def8901") });
Soft Deletes
Instead of removing documents, sometimes apps just mark them as deleted:
db.students.updateOne({ name: "Zara" }, { $set: { deleted: true } });
Then you can filter it out in your queries:
db.students.find({ deleted: { $ne: true } });
Query Optimization
To test these example we need to import a data file using mongoimport which comes from the Database Tools found here:
Then add the path to System Variables under Path click new and put the bin folder path inside the Tools folder
Import a File
mongoimport --db mongo_learn --collection students --file "C:\Users\snowfox\Downloads\students_large_dataset.json" --jsonArray
Explanation:
mongoimport | Command-line tool comes with MongoDB, used to load data from a file into a MongoDB collection |
—db | Name of database to import into |
—collection | Name of collection to create or insert into |
—file | Path to input file (JSON, CSV, or TSV) |
If file looks like this then use —jsonArray:
[
{ "name": "Ali", "age": 22 },
{ "name": "Zara", "age": 24 }
]
Otherwise omit if it looks like this:
{ "name": "Ali", "age": 22 }
{ "name": "Zara", "age": 24 }